Level
Beginner
Course Fee
USD 65
Age Group
12+ Years
Prerequisite
You should be already comfortable creating static HTML templates and styling them using CSS. If you don't know how to do that, then please enroll in our Intro to Web Development Course first.
Description
We have designed this learning path to take you from a complete beginner to a proficient JavaScript developer. This course is meticulously structured to equip you with the essential skills needed to thrive in modern web development.
The course consists of a single comprehensive module, divided into 11 lessons that progressively build on your knowledge. Each lesson is designed to provide a seamless learning experience, helping you transition smoothly from foundational concepts to advanced techniques.
Course Features
Learn the fundamentals of JavaScript from scratch.
Master control flow and functions for effective programming.
Understand arrays and objects for managing data structures.
Gain skills in DOM manipulation for dynamic web pages.
Handle user events to create interactive experiences.
Implement asynchronous programming with Fetch API and Axios.
Build real-world projects to solidify your knowledge.
Learn error handling techniques to create robust applications.
Develop a final project that showcases your skills.
Get hands-on experience with practical applications and challenges.
Join us and take your first step toward becoming a skilled JavaScript developer!
Course Outline
Lesson 1: Introduction to JavaScript
Topics:
- Overview of JavaScript:
- History and evolution
- Role in web development
- Basic syntax and structure:
- Variables and data types
- Statements and expressions
- Comments and whitespace
Homework:
- Write a simple script that prompts the user for their name, stores it in a variable, and displays a personalized greeting using alert().
Lesson 2: Variables and Data Types
Topics:
- Declaring variables:
- Using var, let, and const
- Variable naming conventions
- Data types:
- Strings, numbers, booleans
- Type coercion
- Basic operations:
- Arithmetic operations
- String concatenation
Homework:
- Create a program that takes two numbers as input, stores them in variables, and displays the result of their multiplication.
Lesson 3: Control Flow and Loops
Topics:
- Conditional statements:
- if, else if, and else
- Switch statements
- Looping structures:
- for loops
- while loops
- Loop control statements (break and continue)
Homework:
- Write a program that checks if a user's entered number is even or odd and displays a corresponding message.
Lesson 4: Functions
Topics:
- Declaring functions:
- Function expressions vs. function declarations
- Naming conventions
- Function parameters and arguments
- Return statements and function output
Homework:
- Develop a function that calculates the area of a circle given its radius.
Lesson 5: Arrays and Objects
Topics:
- Arrays:
- Creating and initializing arrays
- Accessing and modifying array elements
- Indexing and accessing elements
- Modifying elements: push(), pop(), shift(), unshift()
- Slicing and splicing: slice(), splice()
- Iterating through arrays: forEach(), map(), filter()
- Objects:
- Creating and manipulating objects
- Accessing and modifying object properties
- Dot notation and bracket notation
- Adding and removing properties
- Object methods
Homework:
- Build a program that uses an array of objects to store information about your favorite movies, including title and genre. Implement the following actions:
- Add a new movie to the list using push().
- Remove a movie by its title using filter() or splice().
- Display only movies of a certain genre using filter().
- Update the genre of a specific movie using object property modification.
Lesson 6: DOM Manipulation
Topics:
- Document Object Model (DOM):
- Understanding the DOM tree
- Selecting and manipulating elements
- Changing styles and content:
- Modifying text content
- Changing element styles
Homework:
- Create a simple webpage with a button that, when clicked, changes the text content of a paragraph.
Lesson 7: Events
Topics:
- Handling user events:
- Click events
- Key events
- Event listeners:
- Attaching event listeners to elements
- Responding to user actions
Homework:
- Implement form validation using JavaScript to check if a user has entered a valid email address.
Lesson 8: Fetch API and Asynchronous Programming
Topics:
- Introduction to asynchronous programming
- Fetch API for making asynchronous requests:
- Making GET requests
- Handling JSON responses
Homework:
- Build a small app that fetches and displays data from an external API.
Lesson 9: Error Handling
Topics:
- Understanding and handling errors in JavaScript
- Using try-catch blocks for graceful error handling
Homework:
- Modify a program to include error handling for user input (e.g., non-numeric input).
Lesson 10: ES6 Features
Topics:
- Arrow functions:
- Syntax and benefits
- Template literals:
- Creating dynamic strings
- Destructuring:
- Object and array destructuring
- let and const:
- Block-scoped variables
Homework:
- Refactor previous code, especially functions, using ES6 features where applicable.
Lesson 11 - 12: Project and Review
Topics:
- Combining all learned concepts in a final project
Homework:
- Build a small interactive web application of your choice, applying the skills learned throughout the course.
Why Should You Enroll in this Course?
Learn JavaScript from Scratch – Begin your journey with foundational concepts and progress to advanced techniques.
Control Flow and Functions – Master essential programming constructs, including loops, functions, and error handling.
Arrays and Objects – Discover how to work with data structures that are crucial for any JavaScript application.
DOM Manipulation – Gain the skills to interact with and modify web page elements dynamically.
Events Handling – Learn to respond to user actions and create interactive experiences.
Fetch API and Asynchronous Programming – Understand how to make API requests and handle data asynchronously.
Real-World Projects – Build a complete interactive web application, applying the skills learned throughout the course.
Certificate
Course Certificate: Yes
Eligibility for Certificate: Final Project + 80% attendance
Schedule
Classes per Week: 2
Duration: 6 weeks
Days/Timing: Teacher will decide with the student
Instructor
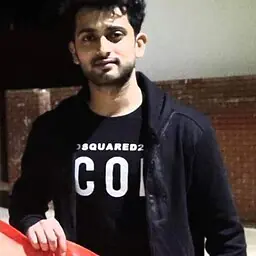